Arduino-Trigonometry Function
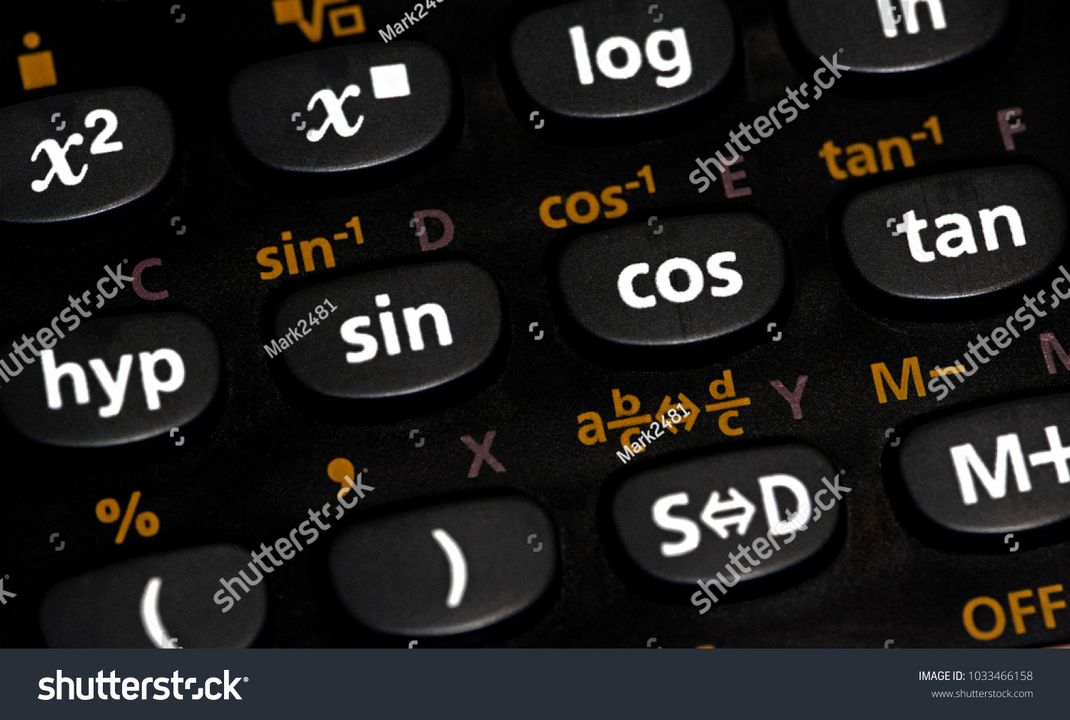
This article details the use of Arduino's Trigonometry function.
The Trigonometry function can be used to calculate cos, sin, and tan.
For other Arduino functions and libraries, please refer to the following article.
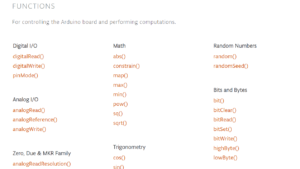
Trigonometry Functions
Functions | Parameters | Returns | Explanation |
---|---|---|---|
double cos(float rad) | rad: The angle in radians(rad) | The cos of the angle | Calculates the cosine of the angle |
double sin(float rad) | rad: The angle in radians(rad) | The sin of the angle | Calculates the sine of the angle |
double tan(float rad) | rad: The angle in radians(rad) | The tan of the angle | Calculates the tangent of the angle |
cos()/Calculates the cosine of the angle
- Function: double cos(float rad)
- Parameter: rad⇒The angle in radians(rad)
- Return: The cos of the angle
The cos() function returns the value calculated by cos-calculating the number specified in the parameter.
sin()/Calculates the sine of the angle
- Function: double sin(float rad)
- Parameter: rad⇒The angle in radians(rad)
- Return: The sin of the angle
The sin() function returns the value calculated by sin-calculating the number specified in the parameter.
tan()/Calculates the tangent of the angle
- Function: double tan(float rad)
- Parameter: rad⇒The angle in radians(rad)
- Return: The tan of the angle
The tan() function returns the value calculated by tan-calculating the number specified in the parameter.
Sample Programs (Sample Sketches)
- Arduino Uno
- USB Cable
- PC(For Program Writing and Serial Monitor)
cos()/Calculates the cosine of the angle
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
double cal;//Declare "cal" as a variable with double
cal = cos(0);//Calculate cos0(cos0°) , Assign 1.00 to cal
Serial.print("cos0: ");//Send string "cos0: "
Serial.println(cal);//Send number "1.00", New line
cal = cos(PI/2);//Calculate cosπ/2(cos90°) , Assign -0.00 to cal
Serial.print("cosπ/2: ");//Send string "cosπ/2: "
Serial.println(cal);//Send number "-0.00", New line
cal = cos(PI);//Calculate cosπ(cos180°) , Assign -1.00 to cal
Serial.print("cosπ: ");//Send string "cosπ: "
Serial.println(cal);//Send number "-1.00", New line
cal = cos(PI*3/2);//Calculate cos3π/2(cos270°) , Assign 0.00 to cal
Serial.print("cos3π/2: ");//Send string "cos3π/2: "
Serial.println(cal);//Send number "0.00", New line
cal = cos(PI*2);//Calculate cos2π(cos360°) , Assign 1.00 to cal
Serial.print("cos2π: ");//Send string "cos2π: "
Serial.println(cal);//Send number "1.00", New line
delay(1000);//Wait 1sec (1000msec)
}
cos0: 1.00
cosπ/2: -0.00
cosπ: -1.00
cos3π/2: 0.00
cos2π: 1.00
cos0: 1.00
cosπ/2: -0.00
cosπ: -1.00
cos3π/2: 0.00
cos2π: 1.00
The sample program calculates cos0, cosπ/2, cosπ, cos3π/2, and cos2π and displays them on the serial monitor. note that the parameter of cos() is in rad units, not angles.
sin()/Calculates the sine of the angle
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
double cal;//Declare "cal" as a variable with double
cal = sin(0);//Calculate sin0(sin0°) , Assign 0.00 to cal
Serial.print("sin0: ");//Send string "sin0: "
Serial.println(cal);//Send number "0.00", New line
cal = sin(PI/2);//Calculate sinπ/2(sin90°) , Assign 1.00 to cal
Serial.print("sinπ/2: ");//Send string "sinπ/2: "
Serial.println(cal);//Send number "1.00", New line
cal = sin(PI);//Calculate sinπ(sin180°) , Assign -0.00 to cal
Serial.print("sinπ: ");//Send string "sinπ: "
Serial.println(cal);//Send number "-0.00", New line
cal = sin(PI*3/2);//Calculate sin3π/2(sin270°) , Assign -1.00 to cal
Serial.print("sin3π/2: ");//Send string "sin3π/2: "
Serial.println(cal);//Send number "-1.00", New line
cal = sin(PI*2);//Calculate sin2π(sin360°) , Assign 0.00 to cal
Serial.print("sin2π: ");//Send string "sin2π: "
Serial.println(cal);//Send number "0.00", New line
delay(1000);//Wait 1sec (1000msec)
}
sin0: 0.00
sinπ/2: 1.00
sinπ: -0.00
sin3π/2: -1.00
sin2π: 0.00
sin0: 0.00
sinπ/2: 1.00
sinπ: -0.00
sin3π/2: -1.00
sin2π: 0.00
The sample program calculates sin0, sinπ/2, sinπ, sin3π/2, and sin2π and displays them on the serial monitor. note that the parameter of sin() is in rad units, not angles.
tan()/Calculates the tangent of the angle
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
double cal;//Declare "cal" as a variable with double
cal = tan(0);//Calculate tan0(tan0°) , Assign 0.00 to cal
Serial.print("tan0: ");//Send string "tan0: "
Serial.println(cal);//Send number "0.00", New line
cal = tan(PI/2);//Calculate tanπ/2(tan90°) , Assign -22877332.00 to cal
Serial.print("tanπ/2: ");//Send string "tanπ/2: "
Serial.println(cal);//Send number "-22877332.00", New line
cal = tan(PI);//Calculate tanπ(tan180°) , Assign 0.00 to cal
Serial.print("tanπ: ");//Send string "tanπ: "
Serial.println(cal);//Send number "0.00", New line
cal = tan(PI*3/2);//Calculate tan3π/2(tan270°) , Assign -83858280.00 to cal
Serial.print("tan3π/2: ");//Send string "tan3π/2: "
Serial.println(cal);//Send number "-83858280.00", New line
cal = tan(PI*2);//Calculate tan2π(tan360°) , Assign 0.00 to cal
Serial.print("tan2π: ");//Send string "tan2π: "
Serial.println(cal);//Send number "0.00", New line
delay(1000);//Wait 1sec (1000msec)
}
tan0: 0.00
tanπ/2: -22877332.00
tanπ: 0.00
tan3π/2: -83858280.00
tan2π: 0.00
tan0: 0.00
tanπ/2: -22877332.00
tanπ: 0.00
tan3π/2: -83858280.00
tan2π: 0.00
The sample program calculates tan0, tanπ/2, tanπ, tan3π/2, and tan2π and displays them on the serial monitor. note that the parameter of sin() is in rad units, not angles.