Arduino-Time Function
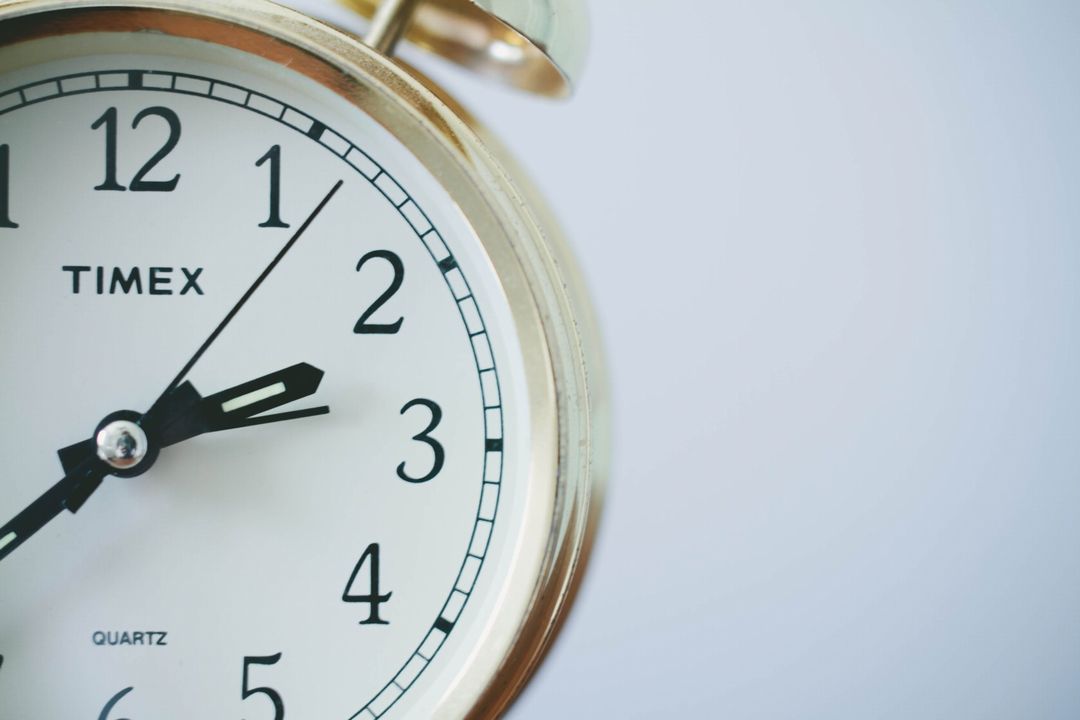
This article details the use of the Arduino Time function.
The Time function can be used to pause the program for the amount of time or to measure the time since running the program.
For other Arduino functions and libraries, please refer to the following article.
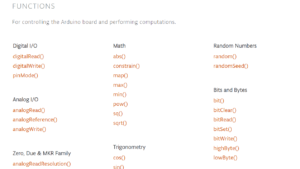
Time Functions
Functions | Parameters | Returns | Explanation |
---|---|---|---|
void delay(unsigned long ms) | ms: Pause time(ms) | None | Pauses the program for the amount of time(ms) |
void delayMicroseconds(unsigned int us) | us: Pause time(us) | None | Pauses the program for the amount of time(us) |
unsigned long millis(void) | None | Number of milliseconds(ms) since the program started | Returns the number of milliseconds(ms) since running the program |
unsigned long micros(void) | None | Number of microseconds(us) since the program started | Returns the number of microseconds(us) since running the program |
delay()/Pauses the program for the amount of time(ms)
- Function: void delay(unsigned long ms)
- Parameter: ms⇒Pause time(ms)
- Return: None
The delay() function pauses the program for the amount of time by setting a wait time (ms: 1/1000 second) as an parameter.
delayMicroseconds()/Pauses the program for the amount of time(us)
- Function: void delayMicroseconds(unsigned int us)
- Parameter: us⇒Pause time(us)
- Return: None
The delayMicroseconds() function pauses the program for the amount of time by setting a wait time (us: 1/1000000 second) as an parameter.
millis()/Returns the number of milliseconds(ms) since running the program
- Function: unsigned long millis(void)
- Parameter: None
- Return: Number of milliseconds(ms) since the program started
The millis() function measures and returns the time (ms: 1/1000 second) since running the program.
micros()/Returns the number of microseconds(us) since running the program
- Function: unsigned long micros(void)
- Parameter: None
- Return: Number of microseconds(us) since the program started
The micros() function measures and returns the time (ms: 1/1000000 second) since running the program.
Sample Programs (Sample Sketches)
- Arduino Uno
- USB Cable
- PC(For Program Writing and Serial Monitor)
delay()/Pauses the program for the amount of time(ms)
void setup() {
pinMode(13, OUTPUT);//Set pin 13 as OUTPUT
}
void loop() {
digitalWrite(13, HIGH);//Pin 13 output set to HIGH (5V)
delay(1000);//Wait 1000msec (1sec)
digitalWrite(13, LOW);//Pin 13 output set to LOW (0V)
delay(1000);//Wait 1000msec (1sec)
}
The sample program waits for the LED on the Arduino Uno board to turn on and off every 1000ms, blinking at 1-second intervals.
delayMicroseconds()/Pauses the program for the amount of time(us)
void setup() {
pinMode(13, OUTPUT);//Set pin 13 as OUTPUT
}
void loop() {
digitalWrite(13, HIGH);//Pin 13 output set to HIGH (5V)
delayMicroseconds(25000);//Wait 25000usec (0.025sec)
delayMicroseconds(25000);//Wait 25000usec (0.025sec)
delayMicroseconds(25000);//Wait 25000usec (0.025sec)
delayMicroseconds(25000);//Wait 25000usec (0.025sec), Total 0.1 sec wait
digitalWrite(13, LOW);//Pin 13 output set to LOW (0V)
delayMicroseconds(25000);//Wait 25000usec (0.025sec)
delayMicroseconds(25000);//Wait 25000usec (0.025sec)
delayMicroseconds(25000);//Wait 25000usec (0.025sec)
delayMicroseconds(25000);//Wait 25000usec (0.025sec), Total 0.1 sec wait
}
The sample program waits for the LED on the Arduino Uno board to turn on and off every 100000us, blinking at 0.1-second intervals.
millis()/Returns the number of milliseconds(ms) since running the program
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned long time;//Declare "time" as a variable with unsigned long
delay(1000);//Wait 1000msec (1sec)
time = millis();//Return time (ms) since running the program
Serial.print(time);//Send time (ms)
Serial.println("ms");//Send string "ms", New line
}
999ms
1999ms
3000ms
3999ms
5000ms
6000ms
7001ms
8001ms
9000ms
10001ms
The sample program repeatedly waits for the program for 1 second, then measures the elapsed time and displays it on the serial monitor.
The numbers displayed on the serial monitor do not add up to exactly every 1000ms because other program execution times are added in addition to delay(1000).
micros()/Returns the number of microseconds(us) since running the program
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned long time;//Declare "time" as a variable with unsigned long
delay(1000);//Wait 1000msec (1sec)
time = micros();//Return time (us) since running the program
Serial.print(time);//Send time (us)
Serial.println("us");//Send string "us", New line
}
1000012us
2000372us
3000732us
4001092us
5001456us
6001816us
7002180us
8002540us
9002908us
10003276us
The sample program repeatedly waits for the program for 1 second, then measures the elapsed time and displays it on the serial monitor.
The numbers displayed on the serial monitor do not add up to exactly every 1000000us because other program execution times are added in addition to delay(1000).