Arduino-How to use Servo Motor
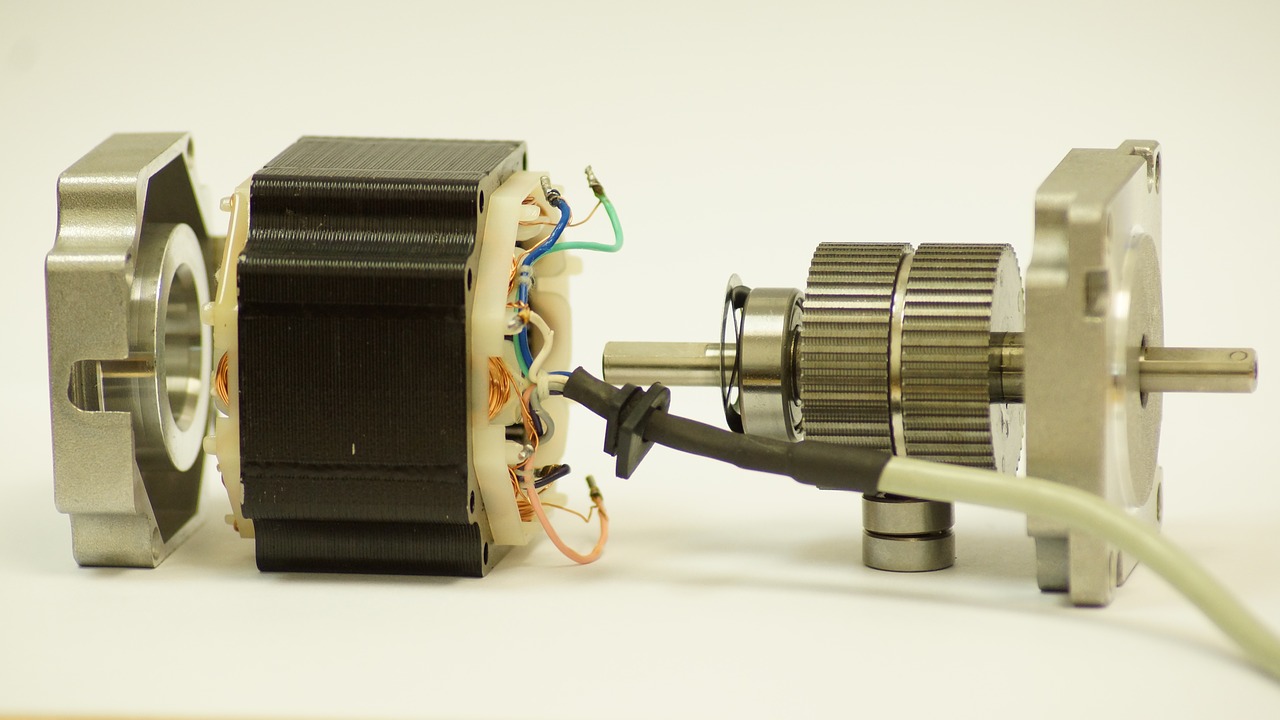
This article details how to use servo motors with Arduino.
The servo motor is operated by a program using "Servo", a library for servo motor control.
For other Arduino functions and libraries, please refer to the following article.
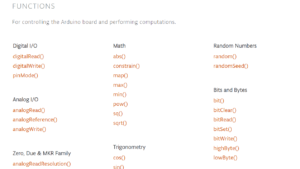
What is Servo Motor?
A servo motor is an electronic component that rotates to a specific angle according to the received pulse width (PWM).
For example, if the pulse width is 0.5 msec, the motor will rotate 0°; if 1.45 msec, 90°; and if 2.4 msec, 180°.
Servo motors are used in various fields such as industrial machinery, industrial robots, and radio-controlled vehicles.
In this article, we will explain a sample program using the SG-90, which integrates a motor, gearbox, and control board and is often used in electronic construction.
Sample Programs (Sample Sketches)
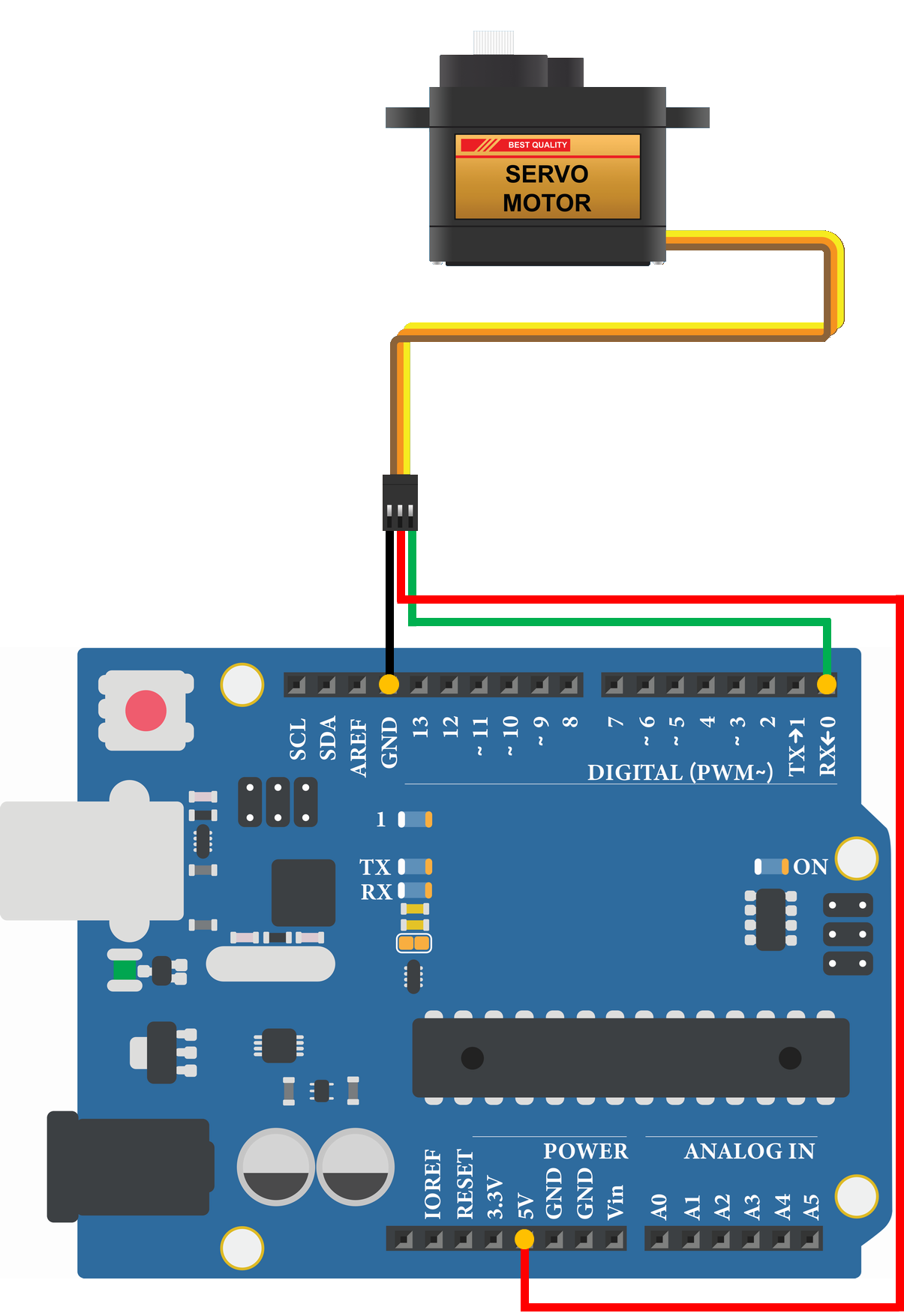
- Servo Motor SG-90
- Arduino Uno
- USB Cable
- Breadboard
- Jumper Wire
- PC(For Program Writing and Serial Monitor)
Move servo motor by specifying angle
#include <Servo.h>
Servo servo5;//Create instance "servo5" for servo motor control
void setup() {
servo5.attach(5);//Assign servo motor control to pin 5
}
void loop() {
servo5.write(0);//Move servo motor to 0° angle
delay(1000);//Wait 1000msec (1sec)
servo5.write(90);//Move servo motor to 90° angle
delay(1000);//Wait 1000msec (1sec)
servo5.write(180);//Move servo motor to 180° angle
delay(1000);//Wait 1000msec (1sec)
servo5.write(90);//Move servo motor to 90° angle
delay(1000);//Wait 1000msec (1sec)
}
In the sample program, the servo motor is operated by specifying an angle. "0°⇒90°⇒180°⇒90°⇒0°…" and rotates every second.
Move servo motor by specifying pulse width
#include <Servo.h>
Servo servo5;//Create instance "servo5" for servo motor control
void setup() {
servo5.attach(5);//Assign servo motor control to pin 5
}
void loop() {
servo5.writeMicroseconds(500);//Pulse width is set to 500 usec, Move servo motor to 0° angle
delay(1000);//Wait 1000msec (1sec)
servo5.writeMicroseconds(1450);//Pulse width is set to 1450 usec, Move servo motor to 90° angle
delay(1000);//Wait 1000msec (1sec)
servo5.writeMicroseconds(2400);//Pulse width is set to 2400 usec, Move servo motor to 180° angle
delay(1000);//Wait 1000msec (1sec)
servo5.writeMicroseconds(1450);//Pulse width is set to 1450 usec, Move servo motor to 90° angle
delay(1000);//Wait 1000msec (1sec)
}
In the sample program, the servo motor is operated by specifying the pulse width. The angle at which the servomotor rotates is determined by the pulse width it receives. 500usec (0.5msec) will move the servomotor to an angle of 0°.
In the sample program, "0°⇒90°⇒180°⇒90°⇒0°…" and rotates every second.
Reads the angle of the servo motor
#include <Servo.h>
Servo servo5;//Create instance "servo5" for servo motor control
void setup() {
servo5.attach(5);//Assign servo motor control to pin 5
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
servo5.write(0);//Move servo motor to 0° angle
Serial.print(servo5.read());//Send "Angle of servo motor (Pin 5)"
Serial.println("°");//Send "°", New line
delay(1000);//Wait 1000msec (1sec)
servo5.write(90);//Move servo motor to 90° angle
Serial.print(servo5.read());//Send "Angle of servo motor (Pin 5)"
Serial.println("°");//Send "°", New line
delay(1000);//Wait 1000msec (1sec)
servo5.write(180);//Move servo motor to 180° angle
Serial.print(servo5.read());//Send "Angle of servo motor (Pin 5)"
Serial.println("°");//Send "°", New line
delay(1000);//Wait 1000msec (1sec)
servo5.write(90);//Move servo motor to 90° angle
Serial.print(servo5.read());//Send "Angle of servo motor (Pin 5)"
Serial.println("°");//Send "°", New line
delay(1000);//Wait 1000msec (1sec)
}
0°
90°
180°
90°
0°
90°
180°
90°
0°
The sample program displays the current servo motor angle on a serial monitor.
Each time the servomotor is rotated, the angle of the servomotor is read.