Arduino-Random Numbers Function
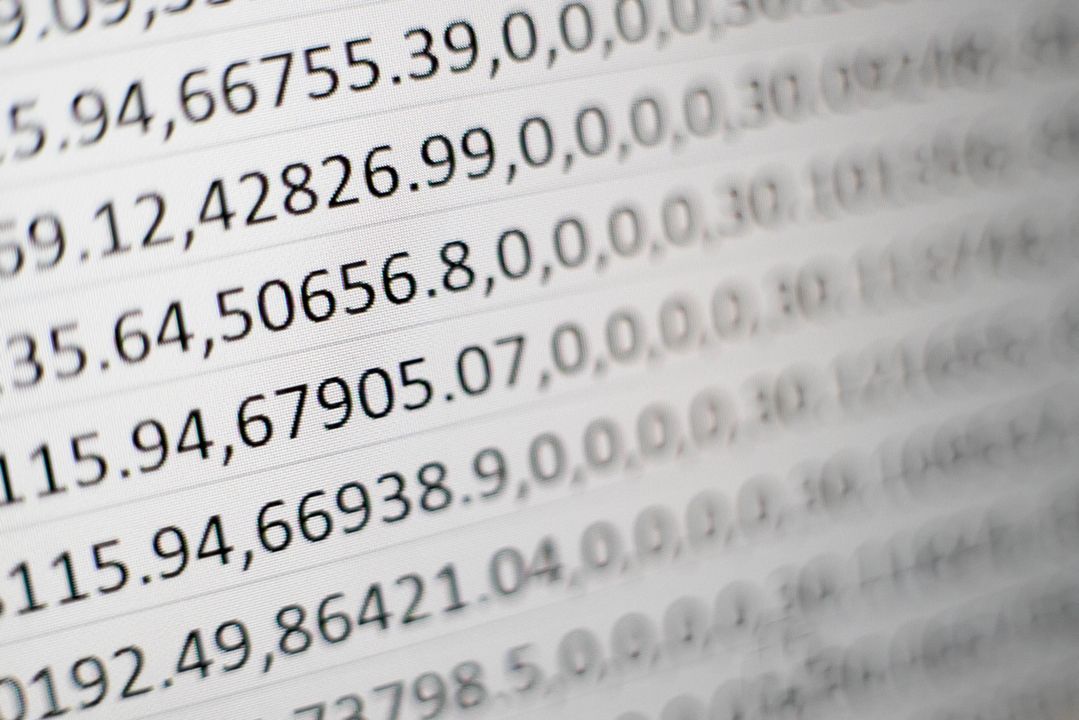
This article details the use of the Arduino's Random Numbers function.
The Random Numbers function can be used to create a random number sequence and obtain random numbers.
For other Arduino functions and libraries, please refer to the following article.
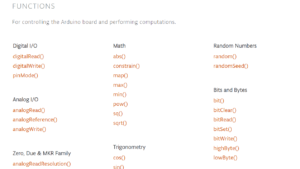
Random Numbers Functions
Functions | Parameters | Returns | Explanation |
---|---|---|---|
void randomSeed(unsigned int seed) | seed: The number to initialize the pseudo-random sequence | None | Initializes the pseudo-random number generator |
long random(long max) long random(long min, long max) | min: The lower value of the random value max: The upper value of the random value | The random value | Generates pseudo-random numbers |
randomSeed()/Initializes the pseudo-random number generator
- Function: void randomSeed(unsigned int seed)
- Parameter: seed⇒The number to initialize the pseudo-random sequence
- Return: None
The randomSeed() function initializes a pseudo-random number generator and takes a random number seed as its parameter. Combined with the random() function, it can be used to generate pseudo-random numbers.
random()/Generates pseudo-random numbers
- Function: long random(long max)/long random(long min, long max)
- Parameter: min⇒The lower value of the random value
- : max⇒The upper value of the random value
- Return: The random value
The random() function returns pseudo-random numbers generated after setting the seed of the random number with the randomSeed() function and setting the minimum and maximum values of the random number as parameters.
Sample Programs (Sample Sketches)
- Arduino Uno
- USB Cable
- PC(For Program Writing and Serial Monitor)
randomSeed()/Initializes the pseudo-random number generator
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
randomSeed(100);//Set the number of random seed to 100
}
void loop() {
long RandomNumber;//Declare "RandomNumber" as a variable with long
RandomNumber = random(300);//Set the maximum value of random numbers to 300
Serial.println(RandomNumber);//Send random number, New line
delay(1000);//Wait 1sec (1000msec)
}
100
189
275
185
209
113
212
151
151
229
In the sample program, a random number sequence is created by setting the random seed to 300, and the random numbers are displayed on the serial monitor. Note that if the random seed is the same value, the same random number sequence will be created, so there is a possibility of obtaining similar random numbers.
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
randomSeed(analogRead(0));//Set the number of random seed to the unconnected analog input pin 0
}
void loop() {
long RandomNumber;//Declare "RandomNumber" as a variable with long
RandomNumber = random(300);//Set the maximum value of random numbers to 300
Serial.println(RandomNumber);//Send random number, New line
delay(1000);//Wait 1sec (1000msec)
}
275
40
238
32
28
229
107
214
139
166
191
299
85
271
250
106
179
54
39
75
By setting the random seed to an unconnected analog input pin, a different random number sequence can be created each time, since the value will be from completely random noise.
In the sample program, we used the unconnected analog input pin 0 as the random seed, and displayed the random numbers on the serial monitor.
By resetting the program with the reset button on the Arduino board, we tried to generate random numbers from two random number sequences (serial monitor pattern 1 and pattern 2), respectively.
random()/Generates pseudo-random numbers
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
randomSeed(100);//Set the number of random seed to 100
}
void loop() {
long RandomNumber;//Declare "RandomNumber" as a variable with long
RandomNumber = random(200, 300);//Set the minimum and maximum random number values to 200 and 300, respectively
Serial.println(RandomNumber);//Send random number, New line
delay(1000);//Wait 1sec (1000msec)
}
200
289
275
285
209
213
212
251
251
229
As already mentioned in the "randomSeed()/Initializes the pseudo-random number generator" sample program, random() can be used to set a minimum and maximum random number in addition to setting only the maximum random number.
In the sample program, the minimum and maximum values of random() random numbers are set to 200 and 300, respectively, so the serial monitor will display random numbers between 200 and 300.