Arduino-Math Function
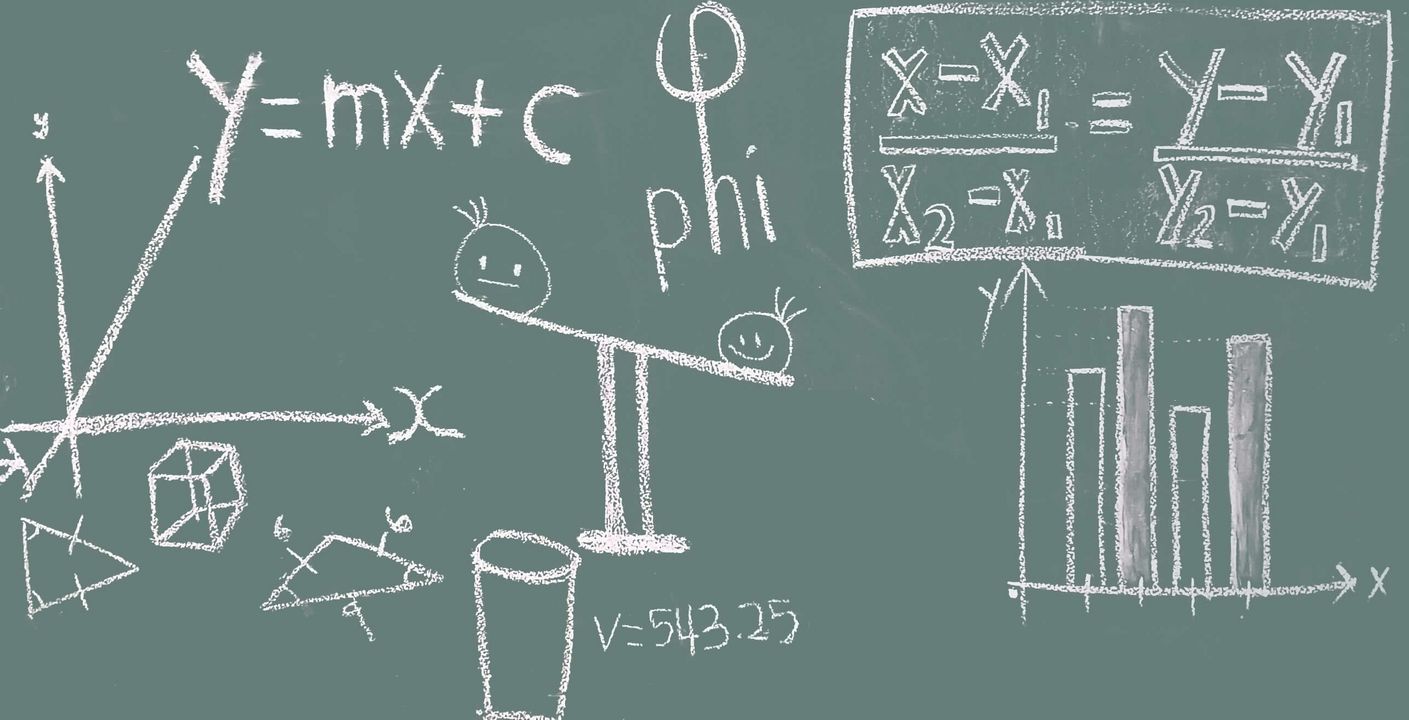
This article details the use of the Arduino Math function.
The Math function can be used to compare numbers, calculate absolute values, powers of magnitude, square roots, and more.
For other Arduino functions and libraries, please refer to the following article.
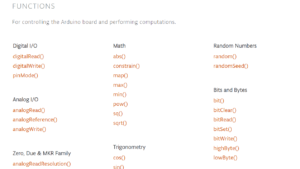
Math Functions
Functions | Parameters | Returns | Explanation |
---|---|---|---|
min(x, y) | x: The first number y: The second number | The smaller of the two numbers | Calculates the minimum of two numbers |
max(x, y) | x: The first number y: The second number | The bigger of the two numbers | Calculates the maximum of two numbers |
abs(x) | x: The number | The absolute value | Calculates the absolute value of the number |
constrain(x, a, b) | x: The number to constrain a: Minimum value of the range b: Maximum value of the range | The value in the range | Returns the number in the range (Minimum or maximum value of the range if outside the range) |
long map(long value, long fromLow, long fromHigh, long toLow, long toHigh) | value: The number to map fromLow: The lower value of the current range fromHigh: The upper value of the current range toLow: The lower value of the target range toHigh: The upper value of the target range | The mapped value | Re-maps the number from one range to another |
double pow(float base, float exponent) | base: The base number exponent: The multiplier number | The value of the power multiplied by | Calculates the value of the number raised to the power |
double sqrt(x) | x: The number | The value of the square root | Calculates the square root of the number |
sq(x) | x: The number | The value of the square for the number | Calculates the square of the number |
min()/Calculates the minimum of two numbers
- Function: min(x, y)
- Parameter: x⇒The first number
- : y⇒The second number
- Return: The smaller of the two numbers
The min() function compares two numbers specified in its parameters and returns the smaller number.
max()/Calculates the maximum of two numbers
- Function: max(x, y)
- Parameter: x⇒The first number
- : y⇒The second number
- Return: The bigger of the two numbers
The max() function compares two numbers specified in its parameters and returns the larger number.
abs()/Calculates the absolute value of the number
- Function: abs(x)
- Parameter: x⇒The number
- Return: The absolute value
The abs() function returns the absolute value of a number specified by its parameter.
constrain()/Returns the number in the range
- Function: constrain(x, a, b)
- Parameter: x⇒The number to constrain
- : a⇒Minimum value of the range
- : b⇒Maximum value of the range
- Return: The value in the range
The constrain() function returns a value within a range, with the target number and the minimum and maximum values of the range as parameters.
map()/Re-maps the number from one range to another
- Function: long map(long value, long fromLow, long fromHigh, long toLow, long toHigh)
- Parameter: value⇒The number to map
- : fromLow⇒The lower value of the current range
- : fromHigh⇒The upper value of the current range
- : toLow⇒The lower value of the target range
- : toHigh⇒The upper value of the target range
- Return: The mapped value
The map() function converts the target value from the original range to the converted range by a percentage.
pow()/Calculates the value of the number raised to the power
- Function: double pow(float base, float exponent)
- Parameter: base⇒The base number
- : exponent⇒The multiplier number
- Return: The value of the power multiplied by
The pow() function returns a power of a number, with a base and a multiplier specified as parameters.
sqrt()/Calculates the square root of the number
- Function: double sqrt(x)
- Parameter: x⇒The number
- Return: The value of the square root
The sqrt() function returns a value calculated by the square root of the number specified in the parameter.
sq()/Calculates the square of the number
- Function: sq(x)
- Parameter: x⇒The number
- Return: The value of the square for the number
The sq() function returns a value calculated as the square of the number specified in the parameter.
Sample Programs (Sample Sketches)
- Arduino Uno
- USB Cable
- PC(For Program Writing and Serial Monitor)
min()/Calculates the minimum of two numbers
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char cal;//Declare "cal" as a variable with unsigned char
cal = min(5, 3);//Compare 5 and 3, Assign smaller 3 to cal
Serial.print("Minimum: ");//Send string "Minimum: "
Serial.println(cal);//Send number "3" (smaller value), New line
delay(1000);//Wait 1sec (1000msec)
}
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
Minimum: 3
The sample program compares the numbers "5" and "3" and displays the smaller number "3" on the serial monitor.
max()/Calculates the maximum of two numbers
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char cal;//Declare "cal" as a variable with unsigned char
cal = min(5, 3);//Compare 5 and 3, Assign larger 5 to cal
Serial.print("Max: ");//Send string "Max: "
Serial.println(cal);//Send number "5" (larger value), New line
delay(1000);//Wait 1sec (1000msec)
}
Max: 5
Max: 5
Max: 5
Max: 5
Max: 5
Max: 5
Max: 5
Max: 5
Max: 5
Max: 5
The sample program compares the numbers "5" and "3" and displays the larger number "5" on the serial monitor.
abs()/Calculates the absolute value of the number
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int cal;//Declare "cal" as a variable with int
cal = abs(5);//Assign the absolute value of +5 to cal
Serial.print("Absolute Value of +5: ");//Send string "Absolute Value of +5: "
Serial.println(cal);//Send number "5" (absolute value of +5), New line
cal = abs(-5);//Assign the absolute value of -5 to cal
Serial.print("Absolute Value of -5: ");//Send string "Absolute Value of -5: "
Serial.println(cal);//Send number "5" (absolute value of -5), New line
delay(1000);//Wait 1sec (1000msec)
}
Absolute Value of +5: 5
Absolute Value of -5: 5
Absolute Value of +5: 5
Absolute Value of -5: 5
Absolute Value of +5: 5
Absolute Value of -5: 5
Absolute Value of +5: 5
Absolute Value of -5: 5
Absolute Value of +5: 5
Absolute Value of -5: 5
The sample program displays the absolute values "5" for "+5" and "5" for "-5" on the serial monitor.
constrain()/Returns the number in the range
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char cal;//Declare "cal" as a variable with unsigned char
cal = constrain(5, 3, 7);//Since the target value "5" is in the range 3 to 7, Assign 5 to cal
Serial.print("x=5, low=3, high=7: ");//Send string "x=5, low=3, high=7: "
Serial.println(cal);//Send number "5", New line
cal = constrain(1, 3, 7);//Since the target value "1" is smaller than the range 3 to 7, Assign 3 to cal
Serial.print("x=1, low=3, high=7: ");//Send string "x=1, low=3, high=7: "
Serial.println(cal);//Send number "3", New line
cal = constrain(10, 3, 7);//Since the target value "10" is lager than the range 3 to 7, Assign 7 to cal
Serial.print("x=10, low=3, high=7: ");//Send string "x=10, low=3, high=7: "
Serial.println(cal);//Send number "7", New line
delay(1000);//Wait 1sec (1000msec)
}
x=5, low=3, high=7: 5
x=1, low=3, high=7: 3
x=10, low=3, high=7: 7
x=5, low=3, high=7: 5
x=1, low=3, high=7: 3
x=10, low=3, high=7: 7
x=5, low=3, high=7: 5
x=1, low=3, high=7: 3
x=10, low=3, high=7: 7
In the sample program, if the target value is within the range, the value is displayed on the serial monitor as it is, and if the target value is outside the range, the minimum or maximum value of the range value is displayed on the serial monitor.
map()/Re-maps the number from one range to another
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
long cal;//Declare "cal" as a variable with long
cal = map(5, 1, 10, 10, 100);//The target value "5" is 10 times the ratio of the range 10-100 to be converted from the original range 1-10, So assign 50 to cal
Serial.print("x=5 from low=1 from high=10 to low=10 to high=100: ");//Send string "x=5 from low=1 from high=10 to low=10 to high=100: "
Serial.println(cal);//Send number "50", New line
delay(1000);//Wait 1sec (1000msec)
}
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
x=5 from low=1 from high=10 to low=10 to high=100: 50
The sample program displays the target value "5" multiplied by 10, "50", on the serial monitor because the ratio of the original range 1-10 to the conversion target range 10-100 is 10 times.
pow()/Calculates the value of the number raised to the power
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
double cal;//Declare "cal" as a variable with double
cal = pow(2, 3);//Since 2 cubed, Assign 8.00 to cal
Serial.print("2 Cubed: ");//Send string "2 Cubed: "
Serial.println(cal);//Send number "8.00", New line
delay(1000);//Wait 1sec (1000msec)
}
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
2 Cubed: 8.00
The sample program displays "8.00" calculated as the "2 cubed" on the serial monitor.
sqrt()/Calculates the square root of the number
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
double cal;//Declare "cal" as a variable with double
cal = sqrt(4);//Since square root of 4, Assign 2.00 to cal
Serial.print("Square Root of 4: ");//Send string "Square Root of 4: "
Serial.println(cal);//Send number "2.00", New line
cal = sqrt(5);//Since square root of 5, Assign 2.24 to cal
Serial.print("Square Root of 5: ");//Send string "Square Root of 5: "
Serial.println(cal);//Send number "2.24", New line
delay(1000);//Wait 1sec (1000msec)
}
Square Root of 4: 2.00
Square Root of 5: 2.24
Square Root of 4: 2.00
Square Root of 5: 2.24
Square Root of 4: 2.00
Square Root of 5: 2.24
Square Root of 4: 2.00
Square Root of 5: 2.24
Square Root of 4: 2.00
Square Root of 5: 2.24
The sample program displays "2.00" calculated as the "square root of 4" and "2.24" calculated as the "square root of 5" on the serial monitor.
sq()/Calculates the square of the number
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int cal;//Declare "cal" as a variable with int
cal = sq(4);//Since 4 squared, Assign 16 to cal
Serial.print("4 Squared: ");//Send string "4 Squared: "
Serial.println(cal);//Send number "16", New line
cal = sq(5);//Since 5 squared, Assign 25 to cal
Serial.print("5 Squared: ");//Send string "5 Squared: "
Serial.println(cal);//Send number "25", New line
delay(1000);//Wait 1sec (1000msec)
}
4 Squared: 16
5 Squared: 25
4 Squared: 16
5 Squared: 25
4 Squared: 16
5 Squared: 25
4 Squared: 16
5 Squared: 25
4 Squared: 16
5 Squared: 25
The sample program displays "16" calculated as "4 squared" and "25" calculated as "5 squared" on the serial monitor.