Arduino-EEPROM Library
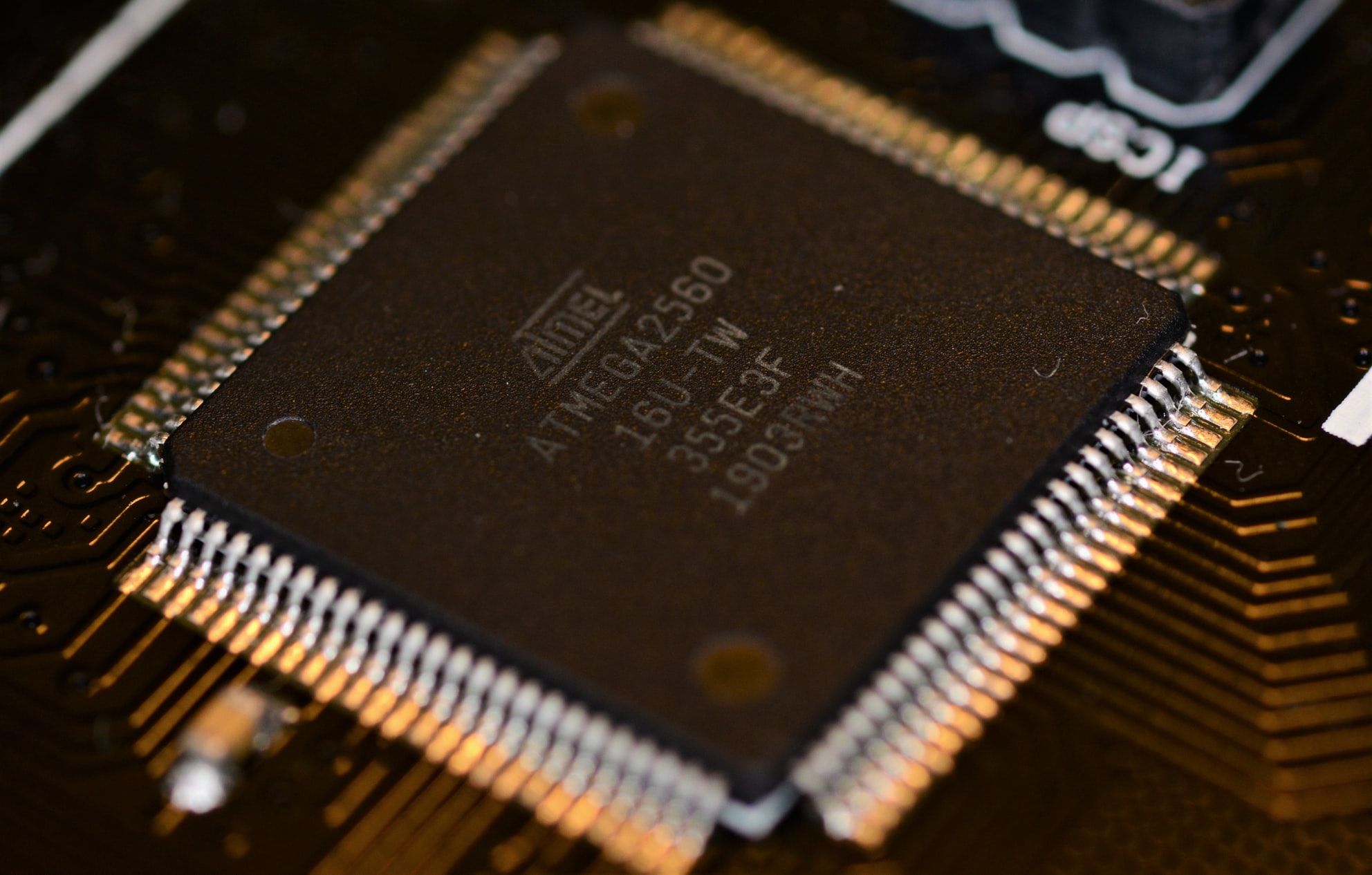
This article details the use of the Arduino EEPROM library.
The Arduino board is equipped with an EEPROM, a non-volatile memory that can retain data even after the power is turned off, and by using the EEPROM library, you can read/write data to/from the EEPROM.
For other Arduino functions and libraries, please refer to the following article.
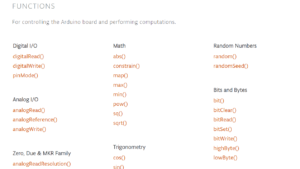
EEPROM Library
Functions | Parameters | Returns | Explanation |
---|---|---|---|
byte Read(int address) | int address: Address | 1Byte data | Reads 1Byte data from the specified address in EEPROM |
void Write(int address, byte value) | int address: Address byte value: 1Byte data | None | Writes 1Byte data at the specified address in EEPROM |
int length(void) | None | EEPROM memory size | Gets the EEPROM memory size |
void Get(int address, T & data) | int address: Address T &data: Data over 2Bytes | None | Gets 2 or more bytes of data from the specified address in EEPROM |
void Put(int address, const T & data) | int address: Address T &data: Data over 2Bytes | None | Writes 2 or more bytes of data at the specified address in EEPROM |
void Update(int address, byte value) | int address: Address byte value: 1Byte data | None | Writes 1Byte data at the specified address in EEPROM; if the original data and the write data are the same, the data is not written. |
byte EEPROM[int address] | int address: Address | 1Byte data | Reads or Writes 1Byte data from the specified address in EEPROM, |
Read()/EEPROM Read
- Function: byte Read(int address)
- Parameter: int address⇒Address
- Return: 1Byte data
The Read() function returns 1-byte data from the EEPROM address specified in the parameter.
Write()/EEPROM Write
- Function: void Write(int address, byte value)
- Parameter: int address⇒Address
- : byte value⇒1Byte data
- Return: None
The Write() function stores the 1-byte data specified by the parameter at the address in the EEPROM specified by the parameter.
length()/Gets the EEPROM memory size
- Function: int length(void)
- Parameter: None
- Return: EEPROM memory size
The length() function returns the EEPROM memory size.
Get()/EEPROM Read 2 bytes or more
- Function: void Get(int address, T &data)
- Parameter: int address⇒Address
- : T & data⇒Data over 2Bytes
- Return: None
The Get() function returns 2 or more bytes of data from the EEPROM address specified in the parameter.
Put()/EEPROM Write 2 bytes or more
- Function: void Put(int address, const T &data)
- Parameter: int address⇒Address
- : T & data⇒Data over 2Bytes
- Return: None
The Put() function stores 2 bytes or more of data specified by the parameter at the address in the EEPROM specified by the parameter.
Update()/EEPROM Update
- Function: void Update(int address, byte value)
- Parameter: int address⇒Address
- : byte value⇒1Byte data
- Return: None
The Update() function stores the 1-byte data specified by the parameter at the EEPROM address specified by the parameter; unlike the Write() function, it does not store the data if the original data is the same as the written data.
EEPROM[]/EEPROM Read or Write
- Function: byte EEPROM[int address]
- Parameter: int address⇒Address
- Return: 1Byte data
EEPROM[] can read or write 1-byte data to a specified address in EEPROM, using a combination of the Read() and Write() functions.
Sample Programs (Sample Sketches)
- Arduino Uno
- USB Cable
- PC(For Program Writing and Serial Monitor)
Read()/EEPROM Read
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int value;
value = EEPROM.read(0x000);//Read data at address "0x000", Assign it to "value"
Serial.print("Address 0x000: ");//Send string "Address 0x000: "
Serial.println(value);//Send "value", New line
while(1);//Infinite loop, Program stop
}
Address 0x000: 255
The sample program reads data at address "0x000" in EEPROM and displays the data on the serial monitor.
Write()/EEPROM Write
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int value;
EEPROM.write(0x000, 59);//Write data "59" at address "0x000"
value = EEPROM.read(0x000);//Read data at address "0x000", Assign it to "value"
Serial.print("Address 0x000: ");//Send string "Address 0x000: "
Serial.println(value);//Send "value", New line
while(1);//Infinite loop, Program stop
}
Address 0x000: 59
The sample program writes data "59" to address "0x000" in EEPROM, then reads the data at address "0x000" in EEPROM and displays the data on the serial monitor.
length()/Gets the EEPROM memory size
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int size;
size = EEPROM.length();//Get EEPROM memory size, Assign to "size"
Serial.print("EEPROM Memory Size: ");//Send string "EEPROM Memory Size: "
Serial.print(size);//Send "size"
Serial.println("Byte");//Send string "Byte", New line
while(1);//Infinite loop, Program stop
}
EEPROM Memory Size: 1024Byte
The sample program gets the memory size of the EEPROM and displays it on the serial monitor.
Get()/EEPROM Read 2 bytes or more
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int value;
EEPROM.put(0x000, 511);//Write data "511" from address "0x000"
EEPROM.get(0x000, value);//Read data at address "0x000", Assign it to "value"
Serial.print("Address 0x000-0x001:");//Send string "Address 0x000-0x001: "
Serial.println(value);//Send "value", New line
while(1);//Infinite loop, Program stop
}
Address 0x000-0x001: 511
In the sample program, the put() function writes two or more bytes of data "511" to the address "0x000" in EEPROM, and then the get() function reads two or more bytes of data "511" at the address "0x000" in EEPROM to display the data on the serial monitor.
Put()/EEPROM Write 2 bytes or more
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int value_1, value_2;
EEPROM.put(0x000, 511);//Write data "511" from address "0x000"
value_1 = EEPROM.read(0x000);//Read data at address "0x000", Assign it to "value_1"
value_2 = EEPROM.read(0x001);//Read data at address "0x001", Assign it to "value_2"
Serial.print("Address 0x000:");//Send string "Address 0x000: "
Serial.println(value_1);//Send "value_1", New line
Serial.print("Address 0x001:");//Send string "Address 0x001: "
Serial.println(value_2);//Send "value_2", New line
while(1);//Infinite loop, Program stop
}
Address 0x000: 255
Address 0x001: 1
In the sample program, the put() function writes two or more bytes of data "511" to address "0x000" in EEPROM, and then the read() function reads the data at addresses "0x000" and "0x001" in EEPROM and displays the data on the serial monitor.
The data written by the put() function will be 2 bytes of data, so it will be written to addresses "0x000" and "0x001".
Therefore, when we read the data with the read() function, we find that 255 (0xFF) of lower-level data is stored at address "0x000" and 1 (0x01) of upper-level data at address "0x01", and when the two data are combined, the result is 511 (0x01FF).
Update()/EEPROM Update
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int value;
EEPROM.update(0x000, 59);//Write data "59" at address "0x000", if the original data is "59", do not write
value = EEPROM.read(0x000);//Read data at address "0x000", Assign it to "value"
Serial.print("Address 0x000:");//Send string "Address 0x000: "
Serial.println(value);//Send "value", New line
while(1);//Infinite loop, Program stop
}
Address 0x000: 59
The sample program writes data "59" to address "0x000" in EEPROM, then reads the data at address "0x000" in EEPROM and displays the data on the serial monitor.
The difference between the update() and write() functions is that if the original data is the same as the written data, it is not stored. In the case of this sample program, if the data already written is "59", no new writing will be done.
EEPROM[]/EEPROM Read or Write
#include <EEPROM.h>
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
int value;
EEPROM[0x000] = 59;//Write data "59" at address "0x000"
value = EEPROM[0x000];//Read data at address "0x000", Assign it to "value"
Serial.print("Address 0x000:");//Send string "Address 0x000: "
Serial.println(value);//Send "value", New line
while(1);//Infinite loop, Program stop
}
Address 0x000: 59
The sample program writes data "59" to address "0x000" in EEPROM, then reads the data at address "0x000" in EEPROM and displays the data on the serial monitor.
EEPROM[] is easy to use because it combines both the Read() and Write() functions to create a more concise program.