Arduino-Bits and Bytes Function
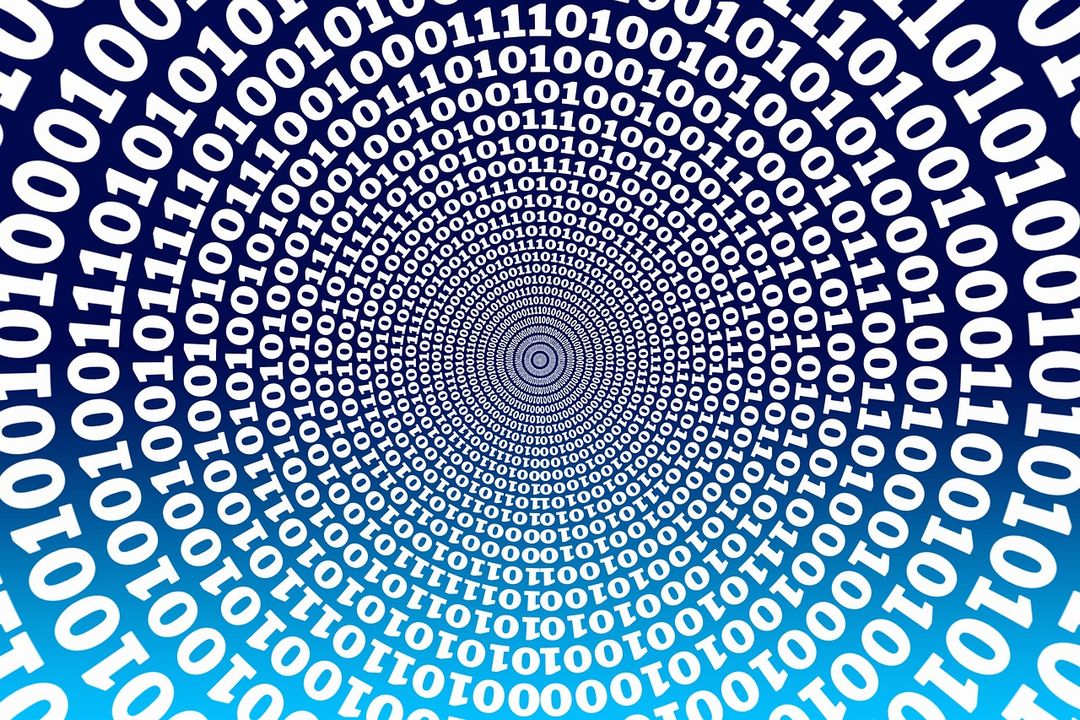
This article details the use of the Arduino's Bits and Bytes function.
The Bits and Bytes function can be used to manipulate bits and bytes.
For other Arduino functions and libraries, please refer to the following article.
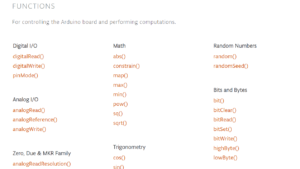
Bits and Bytes Functions
Functions | Parameters | Returns | Explanation |
---|---|---|---|
uint8_t lowByte(x) | x: The value of any type | The low-order Bit | Extracts the low-order Bit |
uint8_t highByte(x) | x: The value of any type | The high-order Bit | Extracts the high-order Bit |
boolean bitRead(x, n) | x: The value to read n: Which Bit to read | The value of the Bit | Reads the Bit of the number |
void bitWrite(x, n, b) | x: The numeric variable to write n: The Bit of the number to write b: The value to write(0 or 1) | None | Writes the Bit of the numeric variable |
void bitSet(x, n) | x: The numeric variable whose Bit to set n: The Bit to set for 1 | None | Sets the Bit of the numeric variable for 1 |
void bitClear(x, n) | x: The numeric variable whose Bit to clear n: The Bit to set for 0 | None | Clears the Bit of the numeric variable for 0 |
bit(n) | n: The Bit whose value to compute | The value of the Bit set to 1 | Computes the value of the specified Bit set to 1 |
lowByte()/Extracts the low-order Bit
- Function: uint8_t lowByte(x)
- Parameter: x⇒The value of any type
- Return: The low-order Bit
The lowByte() function returns the low-order bit of the number specified in the parameter.
highByte()/Extracts the high-order Bit
- Function: uint8_t highByte(x)
- Parameter: x⇒The value of any type
- Return: The high-order Bit
The highByte() function returns the high-order bit of the number specified in the parameter.
bitRead()/Reads the Bit of the number
- Function: boolean bitRead(x, n)
- Parameter: x⇒The value to read
- : n⇒Which Bit to read
- Return: The value of the Bit
The bitRead() function specifies the target number and the digits of the Bit to be read as parameters and returns the Bit read.
bitWrite()/Writes the Bit of the numeric variable
- Function: void bitWrite(x, n, b)
- Parameter: x⇒The numeric variable to write
- : n⇒The Bit of the number to write
- : b⇒The value to write(0 or 1)
- Return: None
The bitWrite() function changes the digit of a Bit to 0 or 1 by specifying the target number, the digit of the Bit, and the number to write to the Bit (0 or 1) as parameters.
bitSet()/Sets the Bit of the numeric variable for 1
- Function: void bitSet(x, n)
- Parameter: x⇒The numeric variable whose Bit to set
- : n⇒The Bit to set for 1
- Return: None
The bitSet() function changes the digit of a Bit to 1 by specifying the target number and the digit of the Bit as parameters.
bitClear()/Clears the Bit of the numeric variable for 0
- Function: void bitClear(x, n)
- Parameter: x⇒The numeric variable whose Bit to clear
- : n⇒The Bit to set for 0
- Return: None
The bitClear() function changes the digit of a Bit to 0 by specifying the target number and the digit of the Bit as parameters.
bit()/Computes the value of the specified Bit set to 1
- Function: bit(n)
- Parameter: n⇒The Bit whose value to compute
- Return: The value of the Bit set to 1
The bit() function computes and returns the value of the digit specified by its parameter, Bit set to 1.
Sample Programs (Sample Sketches)
- Arduino Uno
- USB Cable
- PC(For Program Writing and Serial Monitor)
lowByte()/Extracts the low-order Byte
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char low_byte;//Declare "low_byte" as a variable with unsigned char
low_byte = lowByte(0xF3A1);//Extract the low bits of "0xF3A1", Assign them to "low_byte"
Serial.print("LowByte: ");//Send string "LowByte: "
Serial.println(low_byte, HEX);//Send number "low_byte" in hexadecimal, New line
delay(1000);//Wait 1sec (1000msec)
}
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
LowByte: A1
The sample program specifies 0xF3A1 as the parameter of the lowByte() function and returns 0xA1 of the low bit.
highByte()/Extracts the high-order Byte
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char high_byte;//Declare "high_byte" as a variable with unsigned char
high_byte = highByte(0xF3A1);//Extract the high bits of "0xF3A1", Assign them to "high_byte"
Serial.print("HighByte: ");//Send string "HighByte: "
Serial.println(high_byte, HEX);//Send number "high_byte" in hexadecimal, New line
delay(1000);//Wait 1sec (1000msec)
}
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
HighByte: F3
The sample program specifies 0xF3A1 as the parameter of the highByte() function and returns 0xF3 of the high bit.
bitRead()/Reads the Bit of the number
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char bit1, bit2;//Declare "bit1" and "bit2" as a variable with unsigned char
bit1 = bitRead(0b0101, 0);//Read the 1st digit from "0101" LSB, Assign it to "bit1"
Serial.print("1st digit from 0101 LSB: ");//Send string "1st digit from 0101 LSB: "
Serial.println(bit1);//Send number "bit1" in binary, New line
bit2 = bitRead(0b0101, 3);//Read the 4th digit from "0101" LSB, Assign it to "bit2"
Serial.print("4th digit from 0101 LSB: ");//Send string "4th digit from 0101 LSB: "
Serial.println(bit2);//Send number "bit2" in binary, New line
delay(1000);//Wait 1sec (1000msec)
}
1st digit from 0101 LSB: 1
4th digit from 0101 LSB: 0
1st digit from 0101 LSB: 1
4th digit from 0101 LSB: 0
1st digit from 0101 LSB: 1
4th digit from 0101 LSB: 0
1st digit from 0101 LSB: 1
4th digit from 0101 LSB: 0
1st digit from 0101 LSB: 1
4th digit from 0101 LSB: 0
The sample program reads the specified Bit by the bitRead() function. bit1 reads the 1st digit '1' from the LSB of "0101" and bit2 reads the 4th digit '0' from the LSB of "0101".
bitWrite()/Writes the Bit of the numeric variable
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char bit1=0b1101, bit2=0b1101;//Declare "bit1" and "bit2" as a variable with unsigned char
bitWrite(bit1, 2, 0);//Write "0" to the 3rd digit from LSB of bit1 "1101"
Serial.print("Write '0' to the 3rd digit from LSB of 1101: ");//Send string "Write '0' to the 3rd digit from LSB of 1101: "
Serial.println(bit1, BIN);//Send number "bit1" in binary, New line
bitWrite(bit2, 1, 1);//Write "1" to the 2nd digit from LSB of bit2 "1101"
Serial.print("Write '1' to the 2nd digit from LSB of 1101: ");//Send string "Write '1' to the 2nd digit from LSB of 1101: "
Serial.println(bit2, BIN);//Send number "bit2" in binary, New line
delay(1000);//Wait 1sec (1000msec)
}
Write '0' to the 3rd digit from LSB of 1101: 1001
Write '1' to the 2nd digit from LSB of 1101: 1111
Write '0' to the 3rd digit from LSB of 1101: 1001
Write '1' to the 2nd digit from LSB of 1101: 1111
Write '0' to the 3rd digit from LSB of 1101: 1001
Write '1' to the 2nd digit from LSB of 1101: 1111
Write '0' to the 3rd digit from LSB of 1101: 1001
Write '1' to the 2nd digit from LSB of 1101: 1111
Write '0' to the 3rd digit from LSB of 1101: 1001
Write '1' to the 2nd digit from LSB of 1101: 1111
The sample program writes a specific digit by the bitWrite() function. bit1 writes the 3rd digit from the LSB of "1101" to ' 0' and bit2 writes the 2nd digit from the LSB of "1101" to ' 1'.
bitSet()/Sets the Bit of the numeric variable for 1
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char bit1=0b1101;//Declare "bit1" as a variable with unsigned char
bitSet(bit1, 1);//Set the 2nd digit from LSB of bit1 "1101" to '1'
Serial.print("Set 2nd digit from 1101 LSB to '1': ");//Send string "Set 2nd digit from 1101 LSB to '1': "
Serial.println(bit1, BIN);//Send number "bit1" in binary, New line
delay(1000);//Wait 1sec (1000msec)
}
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
Set 2nd digit from 1101 LSB to '1': 1111
The sample program sets a specific digit to '1' by the bitSet() function. bit1 sets the 2nd digit from the LSB of "1101" to '1'.
bitClear()/Clears the Bit of the numeric variable for 0
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char bit1=0b1101;//Declare "bit1" as a variable with unsigned char
bitClear(bit1, 2);//Clear the 3rd digit from LSB of bit1 "1101" to '0'
Serial.print("Clear 3rd digit from 1101 LSB to '0': ");//Send string "Clear 3rd digit from 1101 LSB to '0': "
Serial.println(bit1, BIN);//Send number "bit1" in binary, New line
delay(1000);//Wait 1sec (1000msec)
}
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
Clear 3rd digit from 1101 LSB to '0': 1001
The sample program clears a specific digit to '0' by the bitClear() function. bit1 clears the 3rd digit from the LSB of "1101" to '0'.
bit()/Computes the value of the specified Bit set to 1
void setup() {
Serial.begin(9600);//Initialize serial communication at 9600bps
}
void loop() {
unsigned char bit1, bit2;//Declare "bit1" and "bit2" as a variable with unsigned char
bit1 = bit(0);//Compute the value of the 1st digit Bit from LSB in binary to '1', Assign it to "bit1"
Serial.print("Compute the value of the 1st digit Bit from LSB to '1': ");//Send string "Compute the value of the 1st digit Bit from LSB to '1': "
Serial.println(bit1, BIN);//Send number "bit1" in binary, New line
bit2 = bit(4);//Compute the value of the 5th digit Bit from LSB in binary to '1', Assign it to "bit2"
Serial.print("Compute the value of the 5th digit Bit from LSB to '1': ");//Send string "Compute the value of the 5th digit Bit from LSB to '1': "
Serial.println(bit2, BIN);//Send number "bit2" in binary, New line
delay(1000);//Wait 1sec (1000msec)
}
Compute the value of the 1st digit Bit from LSB to '1': 1
Compute the value of the 5th digit Bit from LSB to '1': 10000
Compute the value of the 1st digit Bit from LSB to '1': 1
Compute the value of the 5th digit Bit from LSB to '1': 10000
Compute the value of the 1st digit Bit from LSB to '1': 1
Compute the value of the 5th digit Bit from LSB to '1': 10000
Compute the value of the 1st digit Bit from LSB to '1': 1
Compute the value of the 5th digit Bit from LSB to '1': 10000
Compute the value of the 1st digit Bit from LSB to '1': 1
Compute the value of the 5th digit Bit from LSB to '1': 10000
The sample program computes the value "1" of the specified digit Bit by the bit() function. bit1 computes the value "1" of of the 1st digit Bit from LSB, and bit2 computes the value "10000" of the 5th digit Bit from LSB.